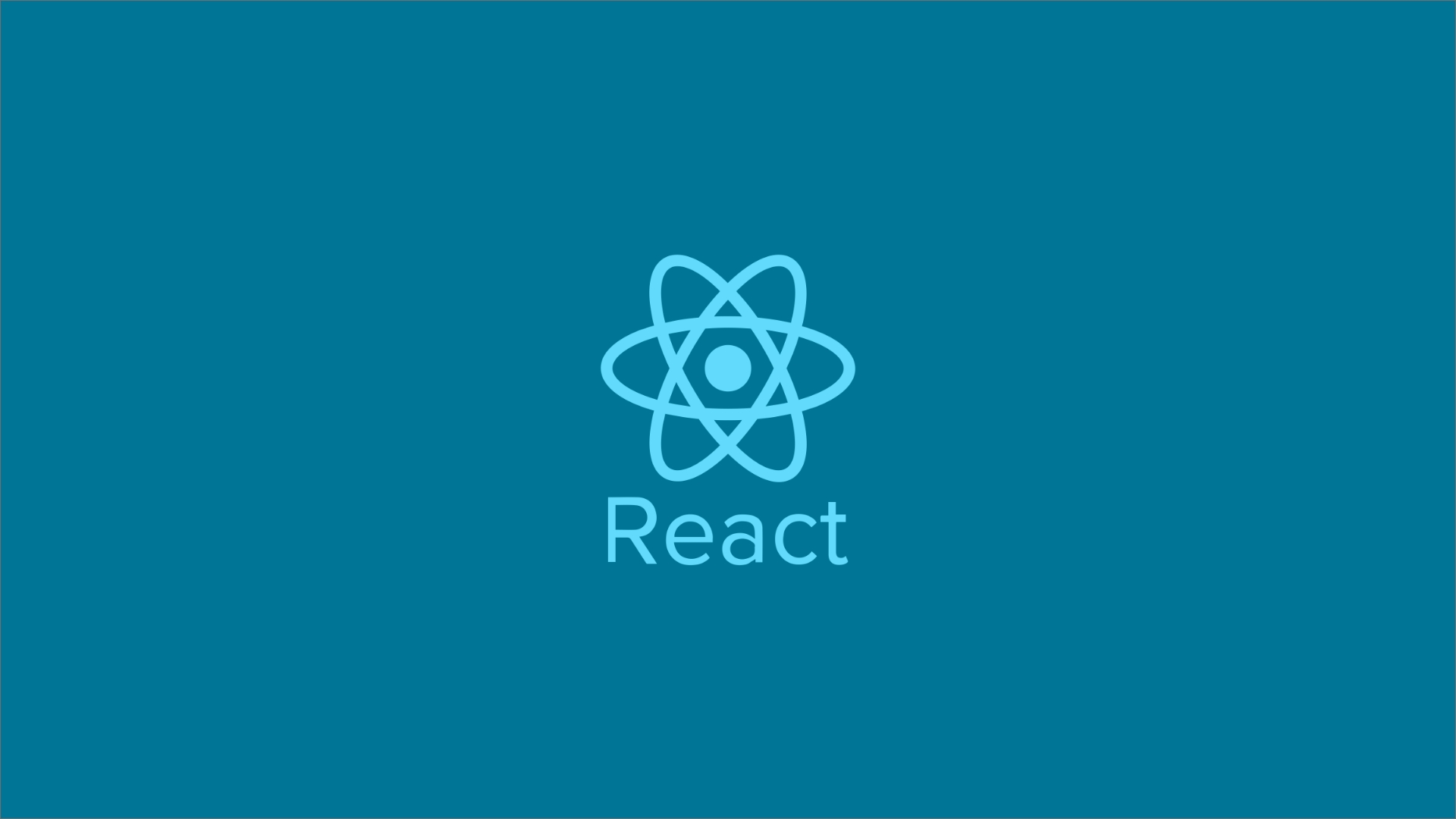
TypeScript Mastery - Creating React Components with Object Props for Enhanced Development
React custom component that receives the object as a prop and then renders the properties of that object
Define Props Interface
Start by defining an interface that represents the structure of the object you want to pass as props. This interface will define the shape of the object and the expected property types.
// Example Props Interface
interface PersonProps {
name: string
age: number
occupation: string
}
Create the React Component
Next, create your React component. You can do this either as a function component or a class component. For this example, we'll create a functional component.
import React from 'react'
// Example Props Interface
interface PersonProps {
name: string
age: number
occupation: string
}
// Functional Component
const PersonComponent: React.FC<PersonProps> = (props) => {
const { name, age, occupation } = props
return (
<div>
<h2>Person Details:</h2>
<p>Name: {name}</p>
<p>Age: {age}</p>
<p>Occupation: {occupation}</p>
</div>
)
}
Using the Component
Now that you have created the component, you can use it in your application and pass an object containing the required properties as props to the component.
import React from 'react'
import PersonComponent from './PersonComponent'
const App = () => {
const personData = {
name: 'John Doe',
age: 30,
occupation: 'Software Engineer'
}
return (
<div>
<h1>My App</h1>
<PersonComponent {...personData} />
</div>
)
}
export default App
In this example, we import the PersonComponent and create an object personData with properties name, age, and occupation. We then use the PersonComponent
and pass the personData object as props using the spread operator (...personData)
. The component will receive the object and render its properties inside the div.
By following these steps, you have successfully created a React component that takes an object as props and displays its properties. This approach allows you to pass complex data structures to your components and make your code more modular and reusable.
Alternative Methods
A generic React component using TypeScript is a component that allows for type flexibility and reusability by using TypeScript generics. Generics enable you to write components that can work with different data types while providing type safety and maintaining proper type inference.
Define Props Interface with Generics
Start by defining a generic interface for the props of your component. Use the TypeScript extends
keyword to specify that the type parameter T
can be any type.
interface GenericComponentProps<T> {
data: T
}
Create the Generic Component
Next, create your generic React component using the interface you defined. You can use TypeScript generics within the component to work with the provided data in a type-safe manner.
import React from 'react'
interface GenericComponentProps<T> {
data: T;
}
const GenericComponent = <T>(props: GenericComponentProps<T>) => {
const { data } = props
return (
<div>
<p>Data: {JSON.stringify(data)}</p>
</div>
)
}
Use the Generic Component
Now you can use the generic component throughout your application by providing the desired data type as a type parameter.
import React from 'react'
import GenericComponent from './GenericComponent'
interface Person {
name: string
age: number
}
interface Product {
id: number
name: string
price: number
}
const App = () => {
const personData: Person = {
name: 'John Doe',
age: 30
}
const productData: Product = {
id: 1,
name: 'Widget',
price: 19.99
}
return (
<div>
<h1>My App</h1>
<GenericComponent data={personData} />
<GenericComponent data={productData} />
</div>
)
}
export default App
In this example, we use the GenericComponent and pass two different data types: Person
and Product
. The generic component automatically adapts to work with the provided data types, ensuring type safety and reusability.
By leveraging TypeScript generics in React components, you can create flexible and versatile components that can handle various data types without compromising on type safety and static type checking. This enhances code readability and maintainability while reducing the likelihood of type-related errors in your React application.
You can explore the demos in this CodePen that I already implemented in here
You can read our other post about React.js UI Libraries in 2023 with CSS-in-JS for Your Portfolios and How To Use Map Function In JavaScript For Rendering A React Component.
Thanks for stopping by and reading this. Cheers!