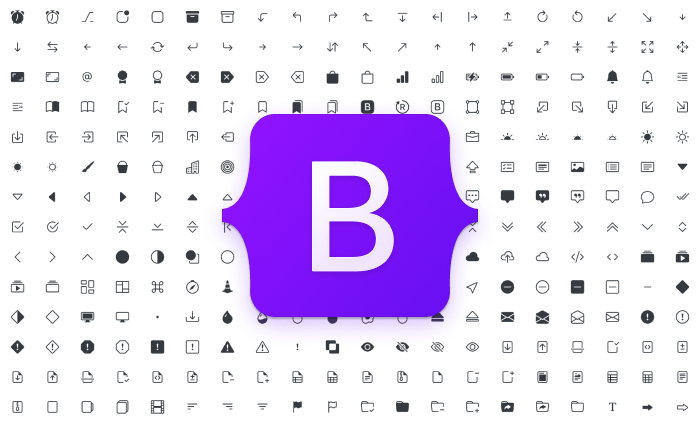
Seamless Integration - Leveraging Bootstrap UI Library in the React.js Ecosystem
Starting a React project with your favorite CSS Framework for your website project using Reactstrap or React Bootstrap library.
Introduction
Bootstrap is a popular CSS framework that provides a comprehensive set of styles and components for building responsive and visually appealing websites and web applications. It includes a grid system, typography, forms, buttons, navigation components, modals, and more. Bootstrap's CSS classes and styles are widely used across the web development community.
Reactstrap and React Bootstrap utilize the existing CSS classes and styles from Bootstrap, but provide a React-specific implementation of the components. This means that you can use the familiar Bootstrap styles and class names, while also benefiting from the component-based nature of React and its associated features such as state management and lifecycle methods.
https://react-bootstrap.netlify.app/
As of now, Bootstrap 5 is the latest stable version available, providing developers with a more modern and streamlined framework. It maintains the core principles of responsiveness, flexibility, and ease of use that Bootstrap is known for.
Why using Bootstrap in React.js?
Bootstrap is a widely adopted CSS framework in website development. Therefore, when developers begin or continue a project with Bootstrap, they will readily recognize its familiar structure and features, enabling them to seamlessly proceed with development.
By building on top of the Bootstrap CSS framework, Reactstrap and React Bootstrap offer React developers a convenient way to incorporate Bootstrap's styling and components into their React projects without the need to manually handle the intricacies of Bootstrap's JavaScript dependencies.
Both Reactstrap and React Bootstrap provide similar functionality and aim to bridge the gap between the Bootstrap framework and React applications. They offer pre-built components that follow Bootstrap's design patterns and can be easily integrated into React projects. The choice between Reactstrap and React Bootstrap often comes down to personal preference or the specific requirements of your project.
Reactstrap and React Bootstrap utilize the existing CSS classes and styles from Bootstrap, but provide a React-specific implementation of the components. This means that you can use the familiar Bootstrap styles and class names, while also benefiting from the component-based nature of React and its associated features such as state management and lifecycle methods.
Container
, Row
, and Col
components in React Bootstrap:
import React from 'react'
import { Container, Row, Col } from 'react-bootstrap'
function App() {
return (
<Container>
<Row>
<Col sm={6}>{/* Content for the left column */}</Col>
<Col sm={6}>{/* Content for the right column */}</Col>
</Row>
</Container>
)
}
export default App
Container
, Row
, and Col
components in Reactstrap:
import React from 'react'
import { Container, Row, Col } from 'reactstrap'
function App() {
return (
<Container>
<Row>
<Col sm="6">{/* Content for the left column */}</Col>
<Col sm="6">{/* Content for the right column */}</Col>
</Row>
</Container>
)
}
export default App
In above examples, we have a row with two columns, each taking up 6 columns on small screens (sm
). To define different column widths for various screen sizes, you can utilize the sm
, md
, lg
, and xl
attributes. Additionally, the offset
prop can be used with both Col
components to create an offset or space between columns. These properties are trademark features commonly found in Bootstrap itself.
If you enjoy working with SCSS/SASS, you can leverage the node-sass
module to integrate SASS node modules into your project. This allows you to organize your SCSS assets directly within your React app's root directory.
Comparison of Reactstrap and React Bootstrap
While Reactstrap and React Bootstrap share a similar goal of integrating Bootstrap components into React applications, there are some differences between them. In terms of functionality, both libraries provide a rich set of components that follow Bootstrap's design patterns. However, the API and component structure may differ slightly. Reactstrap aims to closely mirror Bootstrap's API, making it a great choice for developers already familiar with Bootstrap. On the other hand, React Bootstrap takes advantage of React's component-based nature, providing a more idiomatic React API. Considerations such as performance, ecosystem support, and community engagement may also influence the choice between the two libraries.
Choosing Between Reactstrap and React Bootstrap
When selecting between Reactstrap and React Bootstrap, several factors come into play. The decision often depends on the specific project requirements, team preferences, and familiarity with either library. If you're already well-versed in Bootstrap and prefer an API that closely resembles Bootstrap's, Reactstrap may be the better fit for you. Conversely, if you prioritize a more idiomatic React API that seamlessly integrates with React's ecosystem, React Bootstrap might be the preferred choice. Evaluating the pros and cons of each library, considering the project's needs, and experimenting with both can help in making an informed decision.
Installing
The best way to consume React-Bootstrap and Reacstrap is via the npm package which you can install with npm (or yarn if you prefer).
Install Reactstrap:
npm install reactstrap bootstrap
Install React Bootstrap:
npm install react-bootstrap bootstrap
Both Reactstrap and React Bootstrap allow you to include the Bootstrap CSS by importing it directly into your project:
import 'bootstrap/dist/css/bootstrap.min.css'
Using Boostrap SCSS libraries
If you prefer using the SCSS library, Bootstrap conveniently includes its SCSS library in the node modules installation. To deepen your understanding of this powerful CSS preprocessor, we invite you to read our blog posts on SCSS. Explore here to learn about the benefits and enjoyable aspects of using Sass. Additionally, delve into here to uncover techniques for leveraging SCSS functions, such as for
and each
, to optimize your styling capabilities dynamically and efficiently. To get started, integrate a node SCSS compiler, such as node-sass
, and begin importing and integrating it with your custom SCSS.
Install the node-sass compiler by running the following command:
npm install node-sass
Add the Bootstrap SCSS library to your custom SCSS file, such as ./styles.scss
, and import it into your App.js
or index.js
file:
// Import Bootstrap SCSS files
@import '~bootstrap/scss/functions';
@import '~bootstrap/scss/variables';
@import '~bootstrap/scss/mixins';
// ... import any other Bootstrap SCSS files you need
// Define your custom styles here
.custom-button {
// Your custom button styles
background-color: $primary; // Using Bootstrap's primary color variable
padding: 10px;
border: none;
color: $white;
cursor: pointer;
&:hover {
background-color: darken(
$primary,
10%
); // Darken the primary color on hover
}
}
This will import the Bootstrap SCSS files and include them in your project alongside your custom SCSS styles. In your App.js
or index.js
file, import your custom SCSS file:
import './styles.scss'
By following these steps, you can integrate the node-sass compiler, import the Bootstrap SCSS library, and include your custom SCSS styles in your React.js project. This allows you to combine the power of SCSS and Bootstrap to create customized and visually appealing styles for your application.
Importing components in Reactstrap:
import Button from 'reactstrap'
// or less ideally
import { Button } from 'reactstrap'
Importing components in React Bootstrap:
import Button from 'react-bootstrap/Button'
// or less ideally
import { Button } from 'react-bootstrap'
Using the components in Reactstrap:
export default (props) => {
return <Button color="danger">Danger!</Button>
}
Using the components in React Bootstrap:
export default (props) => {
return <Button variant="danger">Danger!</Button>
}
Conclusion
By incorporating Bootstrap into your React.js application, you can harness its powerful responsive design capabilities, significantly reduce development time, maintain a consistent and visually appealing React UI libraries, customize the framework to perfectly align with your project's requirements, benefit from an active and supportive community, and seamlessly integrate it with the broader React.js ecosystem. Thank you for taking the time to read this.
Topics
Recent Blog List Content:
Most popular React.js UI Libraries - Exploring Mantine and Material-UI
How To Work With Relationship RESTful API Endpoints In React.js
Archive
Stories
Code Road’s Web Development Story List
Code Road
Material UI Administrator Dashboard with Next.js
Code Road
Nuxt.js and Chakra UI Website Template
Code Road
How To Use Apex Charts in Nuxt.js Web Application Dashboard
Code Road
Nuxt.js Dynamic Sitemap and Feed for Static Websites
Code Road
Nuxt.js SEO Head Component
Code Road
Chart.js in Nuxt.js: How To Implement
Code Road
On-page SEO List to Have in your Nuxt.js Static Website
Code Road
How To Build VueJS Geo Location Weather Application
Code Road
MySQL Docker Container with MySQL Workbench and PhpMyAdmin
Code Road